See also
This manual refers to Codac v1, but a new v2 implementation is currently in progress… an update of this manual will be available soon. See more.
Start a C++ project
Tip
main.cpp
:#include <codac.h>
using namespace std;
using namespace codac;
int main()
{
Tube x(Interval(0,10), 0.01, TFunction("cos(t)+abs(t-5)*[-0.1,0.1]"));
vibes::beginDrawing();
VIBesFigTube fig("My first tube");
fig.add_tube(&x, "x");
fig.show();
vibes::endDrawing();
}
For the compilation of your project, you can use CMake with the following file CMakeLists.txt
that you will add in the same directory:
cmake_minimum_required(VERSION 3.0.2)
project(my_project LANGUAGES CXX)
set(CMAKE_CXX_STANDARD 17)
set(CMAKE_CXX_STANDARD_REQUIRED ON)
# Adding IBEX
# In case you installed IBEX in a local directory, you need
# to specify its path with the CMAKE_PREFIX_PATH option.
# set(CMAKE_PREFIX_PATH "~/ibex-lib/build_install")
find_package(IBEX REQUIRED)
ibex_init_common() # IBEX should have installed this function
message(STATUS "Found IBEX version ${IBEX_VERSION}")
# Adding Eigen3
# In case you installed Eigen3 in a local directory, you need
# to specify its path with the CMAKE_PREFIX_PATH option, e.g.
# set(CMAKE_PREFIX_PATH "~/eigen/build_install")
find_package(Eigen3 REQUIRED NO_MODULE)
message(STATUS "Found Eigen3 version ${Eigen3_VERSION}")
# Adding Codac
# In case you installed Codac in a local directory, you need
# to specify its path with the CMAKE_PREFIX_PATH option.
# set(CMAKE_PREFIX_PATH "~/codac/build_install")
find_package(CODAC REQUIRED)
message(STATUS "Found Codac version ${CODAC_VERSION}")
# Compilation
add_executable(${PROJECT_NAME} main.cpp)
target_compile_options(${PROJECT_NAME} PUBLIC ${CODAC_CXX_FLAGS})
target_include_directories(${PROJECT_NAME} SYSTEM PUBLIC ${CODAC_INCLUDE_DIRS} ${EIGEN3_INCLUDE_DIRS})
target_link_libraries(${PROJECT_NAME} PUBLIC ${CODAC_LIBRARIES} Ibex::ibex ${CODAC_LIBRARIES} Ibex::ibex)
The files main.cpp
and CMakeLists.txt
appear in the same directory:
my_project
├── CMakeLists.txt
└── main.cpp
Custom install directory of IBEX and Codac
If you installed IBEX and/or Codac in a custom directory (instead of the file system such as /usr/local/
under Linux),
then you need to specify the CMAKE_PREFIX_PATH
option, as indicated in the above CMakeLists.txt
file.
Another way is to export the CMAKE_PREFIX_PATH
environment variable. For instance:
export CMAKE_PREFIX_PATH=$CMAKE_PREFIX_PATH:$HOME/ibex-lib/build_install
export CMAKE_PREFIX_PATH=$CMAKE_PREFIX_PATH:$HOME/codac/build_install
The compilation of your project is made by the following command line:
mkdir build -p ; cd build ; cmake .. ; make ; cd ..
Lastly, the project can be run with:
./build/my_project
If everything is well installed on your computer, you should see the following window appear:
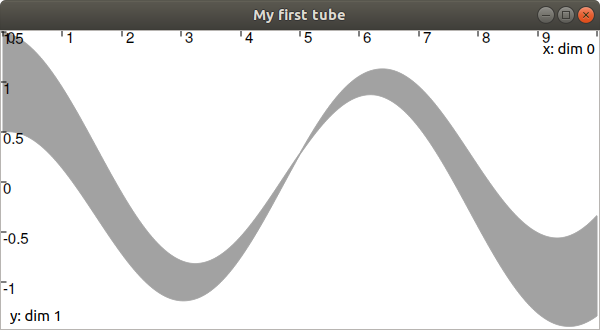